基于SpringBoot的在线教育系统
今天给大家介绍一个基于springboot的在线教育 (opens new window)平台系统,系统是小孟开发的,第一个版本是小锋开发的(小锋的博客 (opens new window)),我进行了本版本的开发。
系统完美运行,无任何的bug,技术较多,可以当做面试的项目或者作为毕设的项目。
目录
# 1. 技术介绍
核心技术:SpringBoot+mybatis;
前端:layui;
开发工具:idea;
数据库:mysql5.7;
模版引擎:thymeleaf (opens new window);
安全框架:SpringSecurity (opens new window);
日志框架:logback;
数据库连接池:druid;
在线编辑器:ckeditor;
图片轮播组件:jQuerySwipeslider;
# 2.功能介绍
本项目分前台用户界面功能和后台管理功能;
前台用户界面功能:
滚动大条幅展示重要通知和课程或者活动;
展示课程,根据实际业务需求,展示课程推荐,最新课程,免费课程,实战课程;
课程搜索,用户输入指定课程关键字,可以搜索查询,也可以根据课程类别分类,和类型进行搜索;
课程详细展示
用户登陆
在线支付
后台管理功能:
管理员登录
课程管理
课程类别管理
用户管理
授课老师管理
订单管理
菜单管理
友情链接管理
系统属性管理
自定义帖子管理
轮转图片帖子管理
# 3. 前端
# 3.1 首页
# 3.2 课程
提供按照课程的类别,类型以及搜索框进行快速查询相关课程
点击任意一门课程,免费课程可以直接观看,vip课程则需要通过支付宝或者微信缴费开通vip进行观看
# 3.3 登入
学习课程时候需要登录才能观看相关视频资料
登入后可以查看个人中心的相关功能
在我的订单界面可以查看已经购买的课程
# 3.4 商品兑换
# 3.5 课程发布
在课程发布页面可以提交发布的课程资料
在我的发布页面可以查看所有已经发布的课程相关信息,查看审核状态
# 4. 后端
# 4.1 登录
# 4.2 系统管理
包括用户管理,角色管理,菜单管理,可以查看对应的信息并添加,导入,修改或删除
角色管理界面可以为角色分配权限
# 4.3 课程管理
可以添加课程,对课程进行分类管理:公共课程,专业课程,免费课程等
在类别管理中可以添加课程的分类信息
在审核功能处可以对上传的视频进行审核
# 4.4 教师管理
# 4.5 导航菜单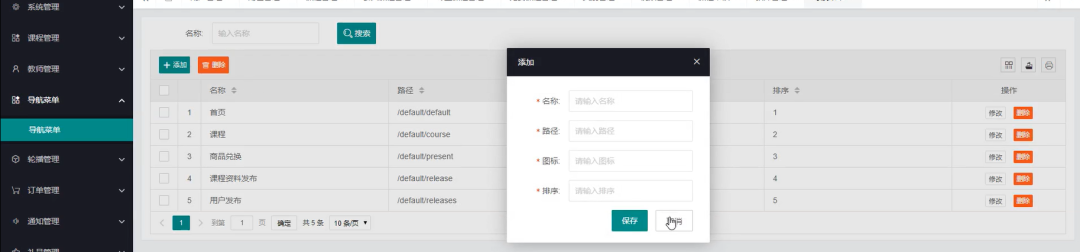
# 4.6 轮播管理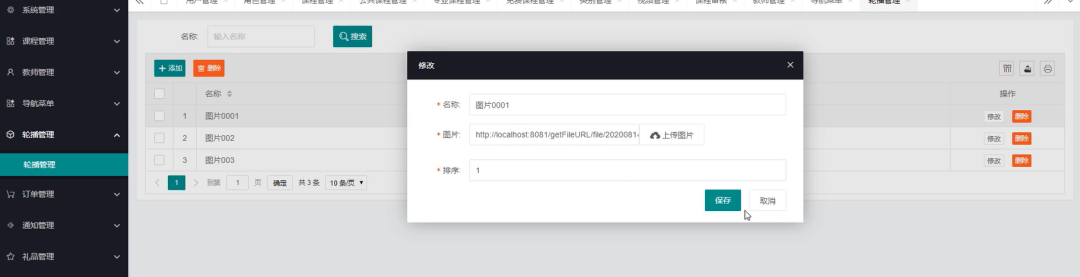
# 4.7 通知管理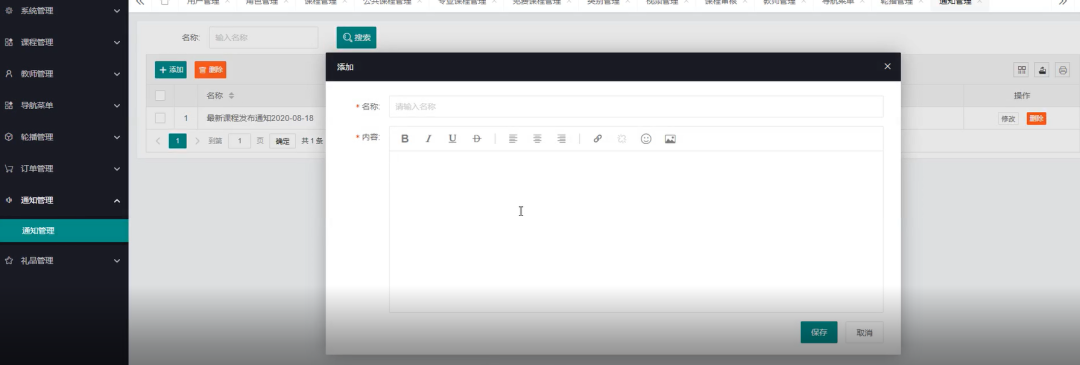
# 4.8 礼品管理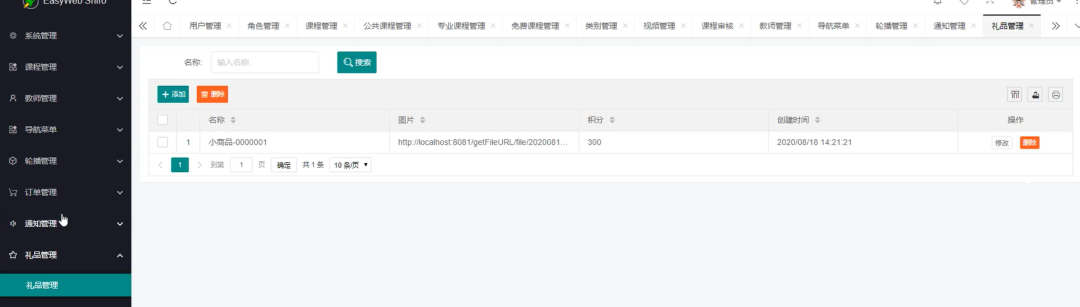
# 5.系统的核心代码
/**
* 操作日志记录注解
* Created by xiaomeng 2020-03-21 17:03
*技术交流v:kaifazixun
* 操作日志记录注解
* Created by wangfan on 2020-03-21 17:03
*/
@Target({ElementType.TYPE, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
public @interface OperLog {
/**
* 模块
*/
String value();
/**
* 功能
*/
String desc();
/**
* 是否记录请求参数
*/
boolean param() default true;
/**
* 是否记录返回结果
*/
boolean result() default false;
}*
*/
@Target({ElementType.TYPE, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
public @interface OperLog {
/**
* 模块
*/
String value();
/**
* 功能
*/
String desc();
/**
* 是否记录请求参数
*/
boolean param() default true;
/**
* 是否记录返回结果
*/
boolean result() default false;
}
@Aspect
@Component
public class OperLogAspect {
private ThreadLocal<Long> startTime = new ThreadLocal<>();
@Autowired
private OperRecordService operRecordService;
@Pointcut("@annotation(com.egao.common.core.annotation.OperLog)")
public void operLog() {
}
@Before("operLog()")
public void doBefore(JoinPoint joinPoint) throws Throwable {
startTime.set(System.currentTimeMillis());
}
@AfterReturning(pointcut = "operLog()", returning = "result")
public void doAfterReturning(JoinPoint joinPoint, Object result) {
saveLog(joinPoint, result, null);
}
@AfterThrowing(value = "operLog()", throwing = "e")
public void doAfterThrowing(JoinPoint joinPoint, Exception e) {
saveLog(joinPoint, null, e);
}
private void saveLog(JoinPoint joinPoint, Object result, Exception e) {
// 获取reques对象
ServletRequestAttributes attributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
HttpServletRequest request = (attributes == null ? null : attributes.getRequest());
// 构建操作日志
OperRecord operRecord = new OperRecord();
operRecord.setUserId(getLoginUserId());
if (startTime.get() != null) operRecord.setSpendTime(System.currentTimeMillis() - startTime.get());
if (request != null) {
operRecord.setRequestMethod(request.getMethod());
operRecord.setUrl(request.getRequestURI());
operRecord.setIp(UserAgentGetter.getIp(request));
}
// 记录异常信息
if (e != null) {
operRecord.setState(1);
operRecord.setComments(StrUtil.sub(e.toString(), 0, 2000));
}
public class BaseController {
/**
* 获取当前登录的user
*/
public User getLoginUser() {
Subject subject = SecurityUtils.getSubject();
if (subject == null) return null;
Object object = subject.getPrincipal();
if (object != null) return (User) object;
return null;
}
/**
* 获取当前登录的userId
*/
public Integer getLoginUserId() {
User loginUser = getLoginUser();
return loginUser == null ? null : loginUser.getUserId();
}
}
/**
* 用户管理
* Created by xiaomeng 2020-12-24 16:10
*技术交流V:kaifazixun
*/
@Controller
@RequestMapping("/sys/user")
public class UserController extends BaseController {
@Autowired
private UserService userService;
@Autowired
private DictionaryDataService dictionaryDataService;
@Autowired
private RoleService roleService;
@Autowired
private OrganizationService organizationService;
@RequiresPermissions("sys:user:view")
@RequestMapping()
public String view(Model model) {
model.addAttribute("sexList", dictionaryDataService.listByDictCode("sex"));
model.addAttribute("organizationTypeList", dictionaryDataService.listByDictCode("organization_type"));
model.addAttribute("rolesJson", JSON.toJSONString(roleService.list()));
return "system/user.html";
}
/**
* 个人中心
*/
@RequestMapping("/info")
public String userInfo(Model model) {
model.addAttribute("user", userService.getFullById(getLoginUserId()));
model.addAttribute("sexList", dictionaryDataService.listByDictCode("sex"));
return "index/user-info.html";
}
/**
* 分页查询用户
*/
@OperLog(value = "用户管理", desc = "分页查询")
@RequiresPermissions("sys:user:list")
@ResponseBody
@RequestMapping("/page")
public PageResult<User> page(HttpServletRequest request) {
PageParam<User> pageParam = new PageParam<>(request);
pageParam.setDefaultOrder(null, new String[]{"create_time"});
return userService.listPage(pageParam);
}
/**
* 查询全部用户
*/
@OperLog(value = "用户管理", desc = "查询全部")
@RequiresPermissions("sys:user:list")
@ResponseBody
@RequestMapping("/list")
public JsonResult list(HttpServletRequest request) {
PageParam<User> pageParam = new PageParam<>(request);
List<User> records = userService.listAll(pageParam.getNoPageParam());
return JsonResult.ok().setData(pageParam.sortRecords(records));
}
/**
* 根据id查询用户
*/
@OperLog(value = "用户管理", desc = "根据id查询")
@RequiresPermissions("sys:user:list")
@ResponseBody
@RequestMapping("/get")
public JsonResult get(Integer id) {
PageParam<User> pageParam = new PageParam<>();
pageParam.put("userId", id);
List<User> records = userService.listAll(pageParam.getNoPageParam());
return JsonResult.ok().setData(pageParam.getOne(records));
}
/**
* 字典管理
* Created by xiaomeng on 2021-03-14 11:29:03
* 技术交流加v:kafazixun
*/
@Controller
@RequestMapping("/sys/dict")
public class DictionaryController extends BaseController {
@Autowired
private DictionaryService dictionaryService;
@RequiresPermissions("sys:dict:view")
@RequestMapping()
public String view() {
return "system/dictionary.html";
}
/**
* 分页查询字典
*/
@OperLog(value = "字典管理", desc = "分页查询")
@RequiresPermissions("sys:dict:list")
@ResponseBody
@RequestMapping("/page")
public PageResult<Dictionary> page(HttpServletRequest request) {
PageParam<Dictionary> pageParam = new PageParam<>(request);
return new PageResult<>(dictionaryService.page(pageParam, pageParam.getWrapper()).getRecords(), pageParam.getTotal());
}
/**
* 查询全部字典
*/
@OperLog(value = "字典管理", desc = "查询全部")
@RequiresPermissions("sys:dict:list")
@ResponseBody
@RequestMapping("/list")
public JsonResult list(HttpServletRequest request) {
PageParam<Dictionary> pageParam = new PageParam<>(request);
return JsonResult.ok().setData(dictionaryService.list(pageParam.getOrderWrapper()));
}
/**
* 根据id查询字典
*/
@OperLog(value = "字典管理", desc = "根据id查询")
@RequiresPermissions("sys:dict:list")
@ResponseBody
@RequestMapping("/get")
public JsonResult get(Integer id) {
return JsonResult.ok().setData(dictionaryService.getById(id));
}
# 6.源码获取
本系统,源码收辛苦费,主要是给大家学习。非诚勿扰,需要加微信:codemeng
或者扫下方二维码